
g(x) - The function that represents the cost of moving from the starting node to a given node.The cost function is calculated as a sum of two other functions: In most cases, this cost function is denoted as f(x). It's also a graph traversal algorithm, however, it's used to handle real-life pathfinding scenarios.Ī* search relies on a knowledge and heuristic cost function for the given node as a way to decide which node it should visit next. Let's take a look at the iterative pseudo-code behind the implementation: dfs(node)Ī* Search is quite different than the two we've previously talked about. Both approaches use a Stack structure in the background, though in the recursive approach, we don't explicitly define it like so. You might've noticed the lack of Stack in this example and I'd like to address it properly. Then goes back to the top, and visits the second neighbor - which advances the search for all of its children, and so on. This means that before advancing to the next neighbor, it visits all of the children of the first neighbor. We then check all of the node's neighbors, and for each one, run the same algorithm if it isn't visited. We start off by calling the algorithm on the given node and set that it's visited. Node set visited true for n in node neighbors Let's take a look at the recursive pseudo-code behind the implementation: dfs(node) The recursive approach is shorter and simpler, though both approaches are pretty much the same performance-wise. You can implement DFS with the help of recursion or iteration.
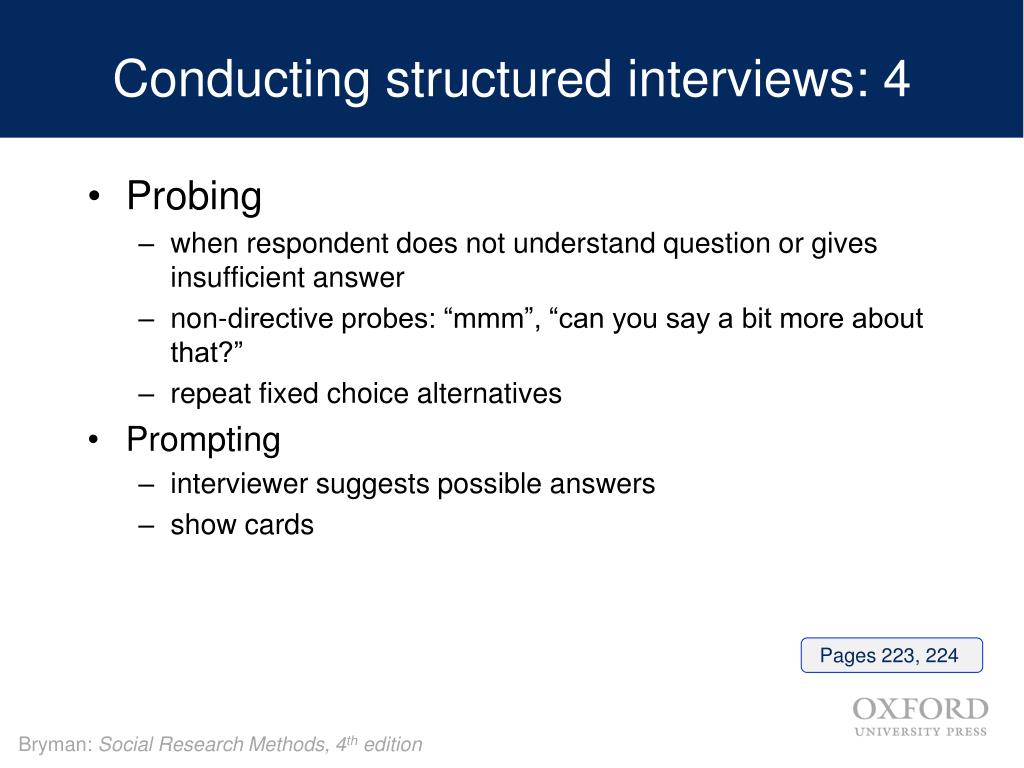
A Stack follows a LIFO (Last-in, first-out) structure, which is the reason it goes as far as possible, before taking the other layers into consideration. To accomplish this, DFS uses a Stack, which is the main difference between these two algorithms. Thanks to this, it's more memory friendly, though not by a lot. The algorithm continues traversing in this fashion until all nodes have been visited and the stack is empty.ĭFS doesn't guarantee to find the shortest possible path and can settle for a local optimum, unlike BFS. Then it backtracks again to the node (5) and since it's already visited nodes (1) and (2), it backtracks to (3) and re-routes to the next branch (8). Once it reaches the final node in that branch (1), it backtracks to the first node where it was faced with a possibility to change course (5) and visits that whole branch, in our case only (2). Depth First Search Interview Question ExampleĬheck if the given graph is strongly connected or not: And probably most noticeably, in your programming career, it's being used for garbage collection. It's being used in your GPS Navigation system to find neighboring locations and even on social networking websites and search engines.īFS found itself used a lot in artificial intelligence and machine learning, especially in robotics. This algorithm is used in a lot of systems all around you! It's very often used for pathfinding, alongside Depth First Search.

And since the queue data structure follows the FIFO (First in, first out) structure, we visit the rest of the children from the second layer, before continuing to visit the children from the proceeding layers. After that, we add the children to the queue.Įach of these children will become the next current node. We then check all of the current node's children and visit them if we haven't visited them before. Since the queue is not empty, we set the current node to be the root node, and remove it from the queue. We start off by adding the starting node to the queue. Let's take a look at the pseudo-code behind the implementation: Queue queue The terminal condition of the algorithm is that the Queue is empty, so it's going to go until it visits every single node. To accomplish this, BFS uses a Queue and this is an important feature of the algorithm. It guarantees to find the shortest possible path, though it pays the price of not being as memory friendly as some other algorithms. It's important to note that BFS will reach the end of the graph and then calculate the shortest path possible. After visiting all the children of the starting node, the algorithm then visits all the children of the already visited children, practically moving to the third layer.
